Understanding and Quantifying Image Noise with Python
- 4 minutes read - 817 words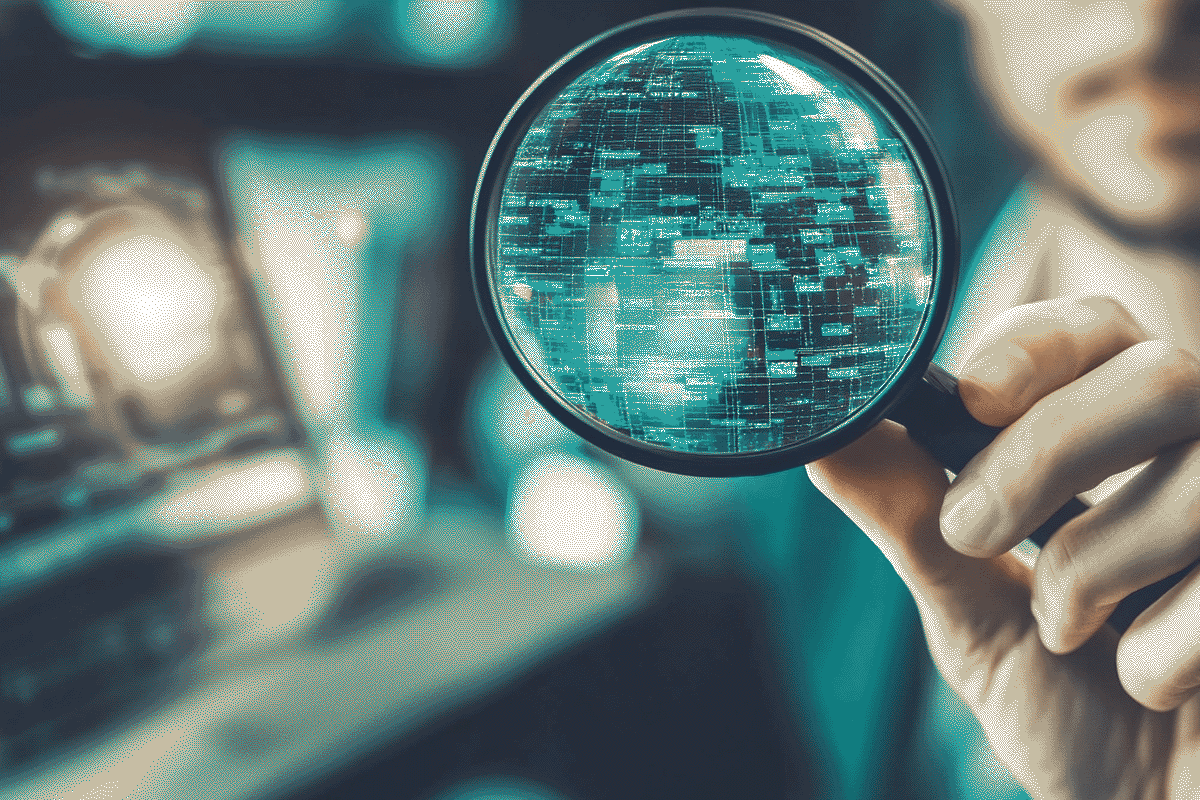
Table of Contents
In the world of image processing, understanding and quantifying noise is crucial for improving image quality. Noise refers to random fluctuations in image data that can degrade the overall quality and accuracy of the image. In this blog post, we will explore a straightforward yet effective method to calculate the mean and standard deviation of noise in an image using OpenCV and Python.
The Code
Below is a Python function that takes an image path as input and returns the mean and standard deviation of the noise in the image. Here’s a breakdown of the code:
import cv2
import numpy as np
def calculate_noise_metrics(image_path):
# Load the image
image = cv2.imread(image_path)
# Convert the image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to the grayscale image
blurred_image = cv2.GaussianBlur(gray_image, (5, 5), 0)
# Calculate the noise by subtracting the blurred image from the original grayscale image
noise = gray_image - blurred_image
# Calculate the mean and standard deviation of the noise
mean_noise = np.mean(noise)
std_noise = np.std(noise)
return mean_noise, std_noise
Code Breakdown
Image Loading: The image is loaded using the
cv2.imread()
function from the OpenCV library. This function reads the image file and returns a numpy array representing the image.Grayscale Conversion: The image is converted to grayscale using the
cv2.cvtColor()
function. This step is necessary because noise analysis is typically performed on grayscale images.Gaussian Blur: A Gaussian blur is applied to the grayscale image using the
cv2.GaussianBlur()
function. This technique reduces noise in images. The function takes three arguments: the source image, the kernel size (a 5x5 kernel in this case), and the standard deviation of the Gaussian distribution.Noise Calculation: Noise in the image is calculated by subtracting the blurred image from the original grayscale image, resulting in a new image that represents the noise.
Noise Metrics: The mean and standard deviation of the noise are calculated using the
np.mean()
andnp.std()
functions from the NumPy library. These metrics provide a quantitative measure of the noise in the image.
Interpretation and Application
The mean and standard deviation of noise in an image help understand the noise level and determine the effectiveness of noise reduction techniques. A higher mean noise value indicates more noise in the image, while a higher standard deviation indicates more variable and less predictable noise.
This code can be applied in various image processing tasks, such as:
- Image Denoising: Noise metrics can help determine the optimal parameters for noise reduction techniques, such as median filtering or wavelet denoising.
- Image Quality Assessment: Noise metrics can assess overall image quality, with higher noise values indicating lower quality.
- Image Enhancement: Noise metrics can guide image enhancement techniques, such as contrast stretching or histogram equalization, to improve overall image quality.
Automated Content Quality Assessment
Incorporating this noise analysis code into a content pipeline for automated content quality assessment can significantly enhance the evaluation process. By automatically calculating the mean and standard deviation of noise in images, the pipeline can identify and flag images with high noise levels, which may indicate lower quality. This automated assessment allows for real-time quality control, ensuring that only high-quality images are used in various applications, such as digital media, marketing, and archival purposes. By integrating noise metrics into the content pipeline, organizations can streamline their quality assurance processes, reduce manual inspection efforts, and maintain high standards for visual content.
Image Quality Assessment based on noise and entropy
Images with a low noise level tend be to be minimalistic examples for low noise , a black image has a very low noise level. This is similar to images with low entropy .
A high noise level is an indicator tha the image has a lot of details and also a high level of complexity, high noise level examples . This is comparable with high entropy .
Noise and Entropy can booth be used to assess image quality and complexity. Measuring entropy and noise is more quantitative than using a LMM or another multi-modal AI for image description.
Measuring noise and entropy of images created with AI is unlike images created with a camera. Measuring noise and entropy of images created with AI, means to measure the quality of the AI engine itself. At least to some extent.
Conclusion
Quantifying noise in images is critical in image processing. The provided code snippet demonstrates a simple yet effective method to calculate the mean and standard deviation of noise in an image using OpenCV and Python. By understanding the noise level, we can make informed decisions about image processing techniques and improve the overall quality and accuracy of the image.