What is System Design, why does it Matter, and how can ChatGPT help?
- 8 minutes read - 1563 words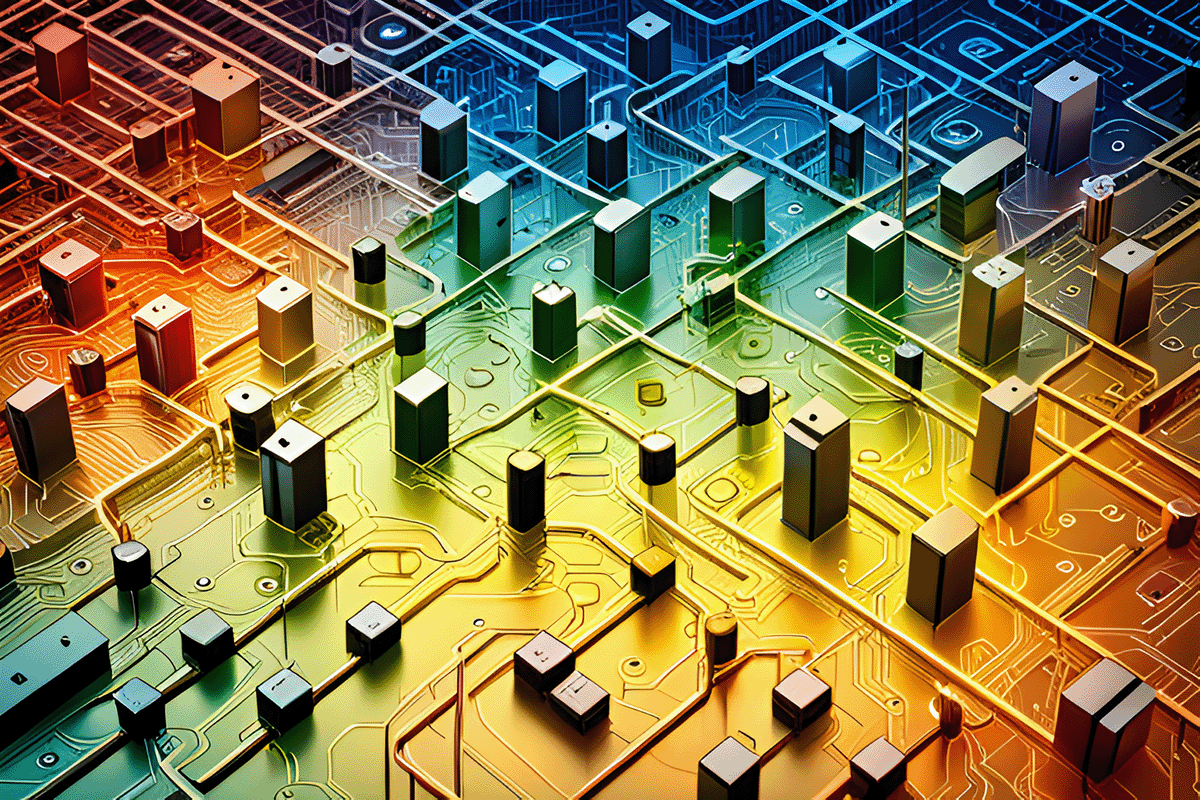
Table of Contents
System design is a fundamental process of creating new systems to solve problems. It’s a broad field that encompasses understanding requirements, defining a problem, creating a solution strategy, and implementing it effectively.
As crucial as it is, system design can often seem daunting, but with a basic understanding and a structured approach, it can be a manageable and rewarding process.
ChatGPT and other LLMs have complex reasoning capabilities; the question is how these capabilities can be used for system design to improve productivity. Create code, analyze system designs, and other activities.
What is System Design?
At its core, system design is about designing the architecture, components, and interfaces for a system. It involves identifying the system’s high-level structure and the discipline to carry out that structure.
The goal is to manage complexity and establish a communication and coordination mechanism among components.
A Simple System Design Example
Let’s dive into a simple system design scenario, which we will call “System-1.” This system has three components: A, B, and C. They interact as follows:
- A affects B such that each time A is triggered, it increases its value by 1, and so does B.
- B affects C, so each time B changes, C decreases by 1.
This simple system demonstrates how different components interact within a system. In this case, triggering A once and letting the system run one iteration, C will decrease by 1.
System Design Interpretation using a prompt
Prompt:
System design setup, called system-1: A -(+1)-> B; B-(-1)->C; initial values: A:0, B:0, C:0; each time A is triggered, it increases the value by 1, which is the value of C after one iteration
Result:
Let’s walk through the system design setup you provided:
You have three components: A, B, and C.
A increases by 1 each time it is triggered.
When A increases, B also increases by 1 (due to the “+1” relationship).
When B increases, C decreases by 1 (due to the “-1” relationship).
All components start at 0.
Based on this setup, let’s see what happens after one iteration:
Trigger A: A becomes 1.
This triggers an increase in B: B becomes 1.
The increase in B triggers a decrease in C: C becomes -1.
So, after one iteration (or one trigger of A), the value of C is -1.
ChatGPT / GPT-4 can also provide Python code for this system.
Get Python code for a system design
Prompt:
Write a Python program with this behavior
Result:
class Node:
def __init__(self, value=0):
self.value = value
def increment(self):
self.value += 1
def decrement(self):
self.value -= 1
# Define the system-1
class System1:
def __init__(self):
self.A = Node(0)
self.B = Node(0)
self.C = Node(0)
def trigger_A(self):
self.A.increment()
self.B.increment()
def trigger_B(self):
self.B.decrement()
self.C.decrement()
def iterate(self):
self.trigger_A()
self.trigger_B()
def print_values(self):
print("Values after one iteration:")
print("A:", self.A.value)
print("B:", self.B.value)
print("C:", self.C.value)
# Run the system-1
system1 = System1()
system1.iterate()
system1.print_values()
Visualizing a system design
You also can create a diagram from a system design.
Prompt:
Visualise the system with a mermaid diagram
Result:
graph LR
A(Node A: 0)
B(Node B: 0)
C(Node C: 0)
A -->|trigger_A| B
B -->|trigger_B| C
B -->|increment| B
C -->|decrement| C
You also can edit the code provided, for example, removing some decrements. Then you can add a new trigger. That means you can design and test a system design interactively and design code for it.
ChatGPT can also run a system design, but the performance is not comparable to Python code. ChatGPT is always probabilistic, approximating the most likely solution; your Python code will be deterministic.
You even can design a system using mermaid code and provide the code to GPT-4, and it will analyze the mermaid code, and you can create Python code.
The Importance of System Design
Understanding the interactions between components, like in our System-1 example, is the essence of system design. It allows us to predict and control the system’s behavior.
System design is not just limited to software or computer systems, but it’s relevant in various fields, such as business processes, team structures, circuit design, and more. It allows us to:
Solve Complex Problems: By breaking down a system into individual components, we can tackle complex problems piece by piece.
Predict System Behavior: Understanding the rules governing system components allows us to predict the system’s overall behavior.
Improve Efficiency: By designing a system carefully, we can optimize performance and efficiency, ensuring that every component interacts as smoothly and effectively as possible.
How to Approach System Design?
Here are some general steps to approach a system design problem:
Understand the System: Clearly define what the system needs to do. What are its inputs and outputs? How do its components interact?
Define the Components: Break down the system into smaller components. What are their responsibilities? How do they interact with each other?
Design the Interactions: Determine how the components will communicate. How does a change in one component affect the others?
Iterate on the Design: Refine the components and their relationships based on the interactions. Make necessary adjustments to improve efficiency.
Implement the System: Once you’re satisfied with the design, implement it. Always be open to revisiting and revising the design as needed.
In conclusion, system design is a crucial skill in many fields. It allows us to tackle complex problems by understanding how different components interact. With a clear understanding and a systematic approach, anyone can master the art of system design.
Principles Good System Design
Some fundamental principles of sound system design include:
- Modularity: Break the system into discrete components with clear interfaces and module connections. This makes the system easier to develop, test, maintain, and scale over time. Modularity allows different teams to work independently on other modules that will integrate.
- Abstraction: Define transparent system interfaces that hide the implementation details of modules or layers. This makes the system more flexible to changes without impacting its usage. Abstraction helps simplify interactions between components and only exposes what other modules need to know.
- Resilience: Design the system to prevent single points of failure and handle errors or outages with minimal impact. This includes having redundancy built-in, handling edge and failure cases, and planning disaster recovery scenarios. Resilient systems are fault-tolerant and designed to avoid downtime.
- Scalability: Ensure the system can handle increased load and demand over time. Plan for how to scale components independently. Use load balancers and caching to distribute demand and decouple systems. Choose architectures that can scale out and scale up to meet future needs.
- Maintainability: The system should be designed for easy troubleshooting, updating, and continued improvement over its lifetime. Use standard interfaces, thorough documentation, version control, and automated testing to maximize maintainability—plan deployment processes to allow seamless updates with minimal disruption.
- Security: Incorporate security controls and best practices into the design to protect against threats and vulnerabilities. Use encryption, authentication, access control lists, threat modeling, and input validation to shield the system and its data. Design for security from the beginning, not as an afterthought.
What capabilities ChatGPT has to enable system design
ChatGPT uses several natural language processing (NLP) tasks to understand and respond to user inputs when simulating a system design. Here are some of the most relevant tasks:
- Named Entity Recognition (NER): This task involves identifying and categorizing critical information in the text, such as names of entities (e.g., A, B, C in your system design setup).
- Text Classification: The model must understand a sentence or paragraph’s overall intent or category. For example, it must classify if a given text describes a system design setup, a specific question about the system, or something else entirely.
- Question Answering: If you ask a question about the system design, the model has to understand the question, find the relevant information, and generate a correct answer.
- Language Generation: After understanding the input and deciding the appropriate response, the model generates a human-like text response. This involves many sub-tasks, including choosing the right words and grammar, maintaining coherence and context, and more.
- Contextual Understanding: The model needs to understand the context throughout the conversation. This includes remembering the system design details, the conversation’s course, and the user’s goals.
- Semantic Parsing: The model needs to understand the meaning and structure of sentences, such as the relationships and actions described in the system design setup.
By applying these and other NLP tasks, ChatGPT can simulate a system design, answer questions about it, create Python code, and interact with users coherently and context-aware.
Conclusions
Taking the time to think through how to architect a robust, modular, and scalable design will pay huge dividends in the success and longevity of any system or solution.
System design is a valuable skill that applies broadly across domains and careers. Spending time to master it is undoubtedly worth the investment.
ChatGPT can support system design and increase your productivity for system design. You can describe a system and its behavior with text, analyze it, and create Python code.
Creating a system design process based on Mermaid code and an interactive system design process looks tempting. Still, GPT-4 as an LLM is unreliable and might create nonworking code.