Using GPT-4 Data Analysis to solve Technical Debt issues
- 4 minutes read - 801 words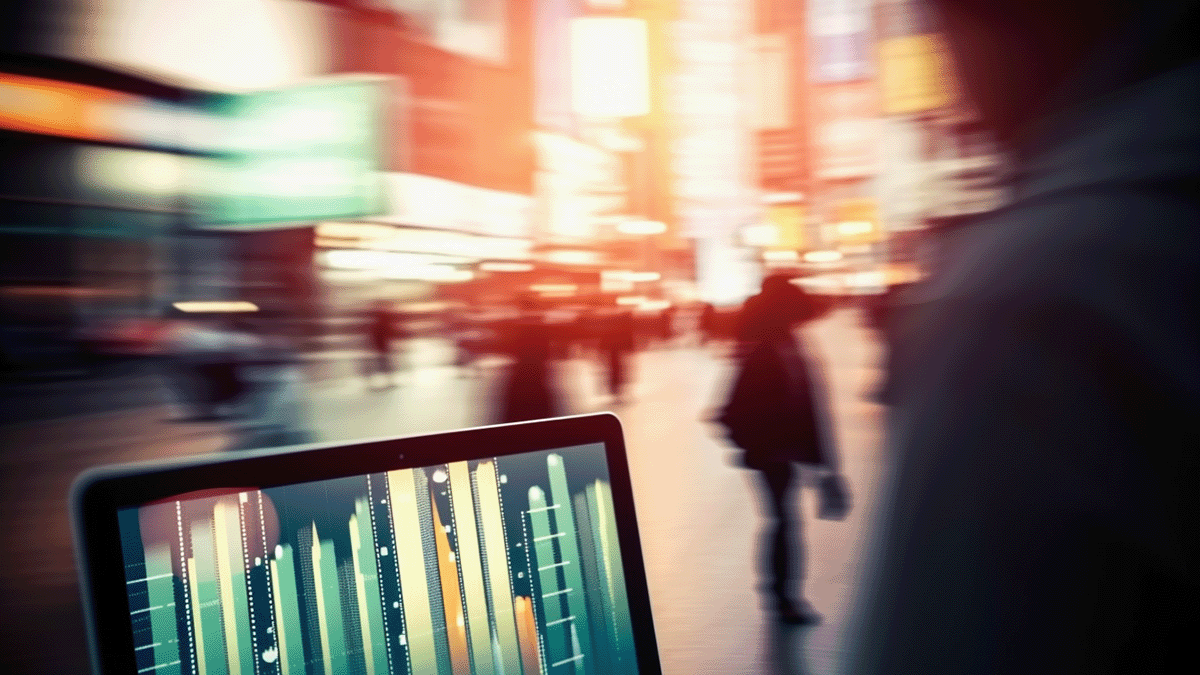
Table of Contents
Legacy systems often form the backbone of many organizations’ IT infrastructure, playing critical roles in core business processes. However, these systems frequently suffer from undocumented data structures accumulated over decades of modifications and patches. This undocumented complexity poses significant challenges during system migrations, especially to modern cloud environments. Large language models (LLMs) like GPT-4 can help address these challenges by analyzing and interpreting undocumented data structures, thereby boosting productivity during legacy system migrations.
The Problem: Undocumented Data Structures
Undocumented data structures in legacy systems can significantly hamper productivity. These structures have evolved, often through ad-hoc modifications and repurposing of existing columns, resulting in complex and opaque data interfaces. The original programmers, who might have deeply understood these structures, have often left the organization, taking their knowledge with them. Consequently, the remaining documentation is incomplete or outdated.
This complexity becomes particularly problematic when migrating these systems to modern cloud environments. A thorough understanding of the existing data structures becomes apparent, as does the necessity for tools that can help decode these structures.
Using GPT-4 for Data Analysis
GPT-4, a state-of-the-art LLM, can analyze and comprehend undocumented data structures, providing valuable insights and assisting in data extraction and transformation. To demonstrate GPT-4’s capabilities, we will use synthetic test data that mimics some of the complexities found in legacy systems.
Workflow:
- Detect Patterns in legacy data samples using a LLM
- Analyse Pattern Recognition
- Derive Python automatically from pattern recognition
- Integrate Python code into the data pipeline
Example Data
Consider the following CSV table:
Key;Flag_1;Flag_2;Payload_1;Payload_2;;
alpha;A;A;100;200;;
beta;A;B;200;beta-001-350;;
gamma;C;B;{C=350};beta-001-450;;
delta;D;C;"(400;A)";{C=950};;
This table contains a mix of standard and non-standard data formats in the Payload_1
and Payload_2
columns. Our goal is to identify dependencies and extract meaningful information from these columns.
Analyzing the Data with GPT-4
Using GPT-4, we can prompt the model to identify dependencies and relationships between the columns:
Prompt:
Given the following CSV table:
Key;Flag_1;Flag_2;Payload_1;Payload_2;;
alpha;A;A;100;200;;
beta;A;B;200;beta-001-350;;
gamma;C;B;{C=350};beta-001-450;;
delta;D;C;"(400;A)";{C=950};;
Identify dependencies between columns Flag_1, Flag_2, Payload_1, Payload_2*
GPT-4’s Analysis:
GPT-4 identifies several dependencies and relationships between the columns:
Flag_1 and Payload_1:
- For rows with
Flag_1
value ‘A’,Payload_1
has a numerical value (100 and 200). - For the row with
Flag_1
value ‘C’,Payload_1
has a non-standard format with curly brackets ({C=350}). - For the row with
Flag_1
value ‘D’,Payload_1
has a non-standard format with parentheses and a semicolon ("(400;A)").
- For rows with
Flag_2 and Payload_2:
- For rows with
Flag_2
value ‘A’,Payload_2
has a numerical value (200). - For rows with
Flag_2
value ‘B’,Payload_2
has a string with a pattern ‘beta-001-xxx’ (beta-001-350 and beta-001-450). - For the row with
Flag_2
value ‘C’,Payload_2
has a non-standard format with curly brackets ({C=950}).
- For rows with
Creating Code to Extract and Transform Data
To further leverage GPT-4, we can generate Python code that extracts and transforms the data, handling non-standard formats and specific patterns.
import csv
import re
from io import StringIO
csv_data = """Key;Flag_1;Flag_2;Payload_1;Payload_2;;
alpha;A;A;100;200;;
beta;A;B;200;beta-001-350;;
gamma;C;B;{C=350};beta-001-450;;
delta;D;C;"(400;A)";{C=950};;"""
# Function to parse the non-standard format
def parse_non_standard(value):
if value.startswith("{") and value.endswith("}"):
return value[1:-1].split("=")[1]
elif value.startswith("(") and value.endswith(")"):
return value[1:-1].split(";")[0]
else:
return value
# Function to parse the 'beta-001-xxx' pattern
def parse_beta_pattern(value):
pattern = r"beta-001-(\d+)"
match = re.match(pattern, value)
if match:
return match.group(1)
else:
return value
# Read CSV data and extract the required columns
csv_reader = csv.reader(StringIO(csv_data), delimiter=";")
result = []
for row in csv_reader:
if len(row) >= 5:
key = row[0]
payload_1 = parse_non_standard(row[3])
payload_2 = parse_non_standard(row[4])
payload_2 = parse_beta_pattern(payload_2)
result.append([key, payload_1, payload_2])
# Print the list
for an item in result:
print(item)
Output
The code reads the given CSV data, processes each row to parse the non-standard formats in the Payload_1
and Payload_2
columns, and extracts the value from the pattern beta-001-xxx
using a regular expression. The result is a list containing the Key
, Payload_1
, and Payload_2
values for each row, with the extracted values included:
['alpha', '100', '200']
['beta', '200', '350']
['gamma', '350', '450']
['delta', '400', '950']
Conclusion
LLMs like GPT-4 can significantly enhance the productivity of data analysts and developers working with legacy systems. By analyzing and recognizing data patterns and correlations, GPT-4 can generate code for data extraction and transformation, simplifying the process of migrating legacy systems to modern environments.
While the synthetic test data used here is more straightforward than real-world legacy systems, the example demonstrates GPT-4’s potential to support system conversion initiatives. However, human oversight remains essential to validate the analysis and ensure the accuracy of the results.
GPT-4’s capabilities can be valuable in addressing technical debt and undocumented complexities in legacy systems, ultimately facilitating smoother and more efficient migrations to modern cloud environments.
Sources:
- https://www.zerone-consulting.com/resources/blog/AI-in-Legacy-Application-Modernization-Opportunities-and-Best-Practices/
- https://www.forbes.com/sites/forbesbusinesscouncil/2024/04/23/modernize-legacy-tech-with-artificial-intelligence-a-field-guide/
- https://eluminoustechnologies.com/blog/impact-of-genai-on-legacy-systems/
- https://www.iese.fraunhofer.de/blog/pattern-recognition/